Creating a route with the Google Maps Directions API
How to create a route between two points with Google Maps.
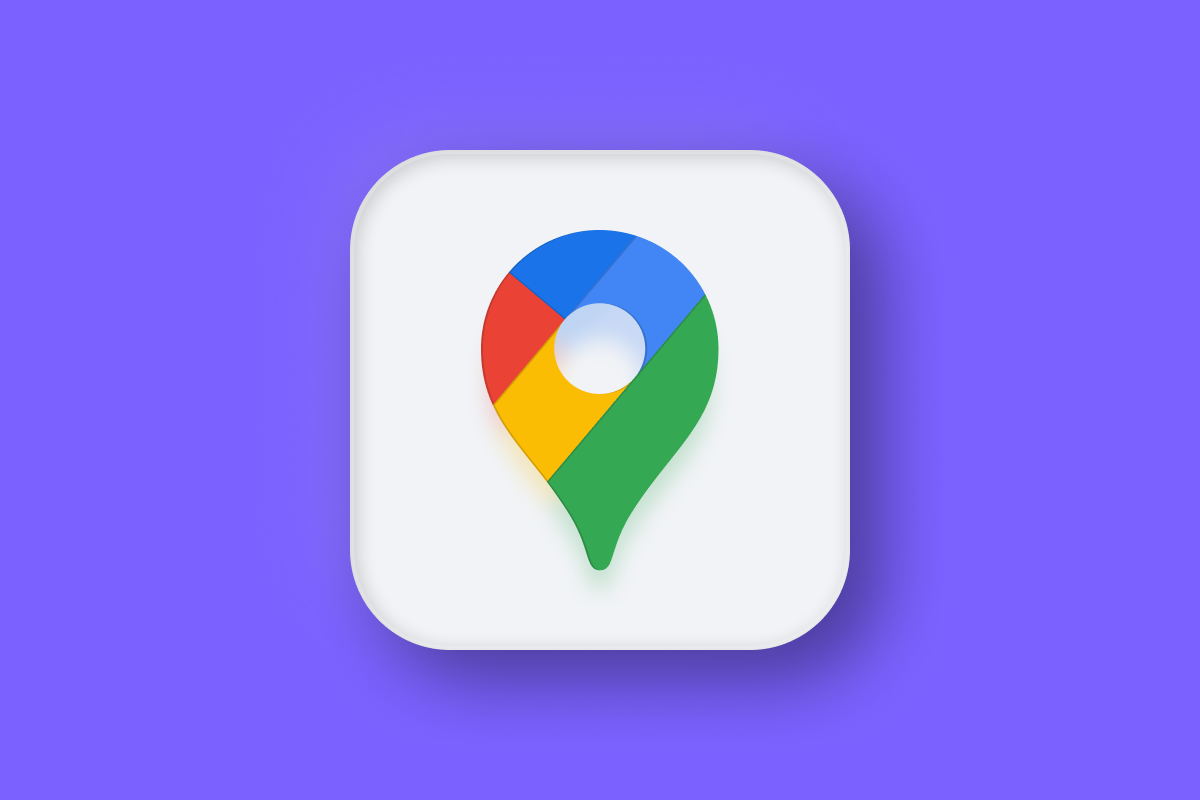
In this tutorial, we'll use the Google Maps Directions API to create a delivery route from a warehouse in downtown San Diego at:
(start) 1337 India St, San Diego, CA 92101, United States
for delivery to:
(A) 1200 Third Ave, San Diego, CA 92101, United States
(B) 707 Tenth Ave, San Diego, CA 92101, United States
(C) 180 Broadway Suite 101, San Diego, CA 92101, United States
(D) 945 Broadway, San Diego, CA 92101, United States
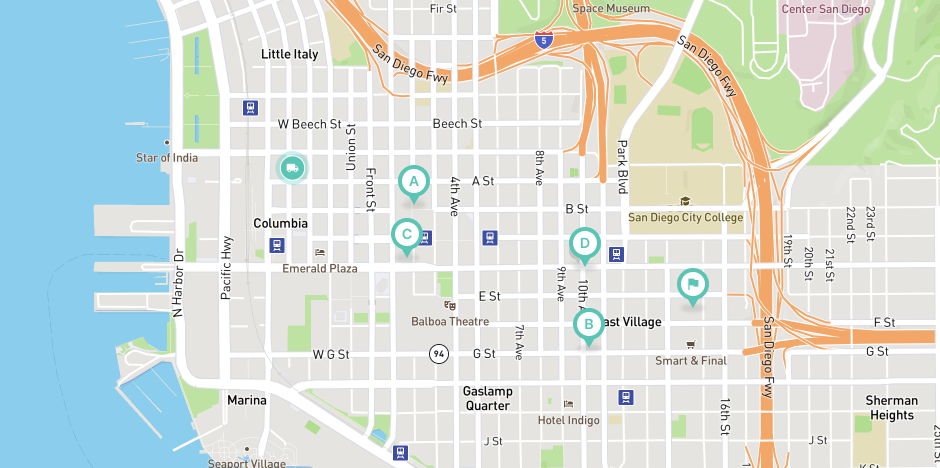
ending at (end) 1485 E St, San Diego, CA 92101, United States. What's the best route to take? Which stops should we visit first? Let's ask Google Maps.
Sign up for a Google Cloud Platform Account
The first thing you need to do is sign up for a Google Cloud Platform (GCP) account, link a credit card and create a project (see this video for instructions). Google Maps can get expensive, but the free $200 monthly credit is more than enough to cover the costs of running this tutorial.
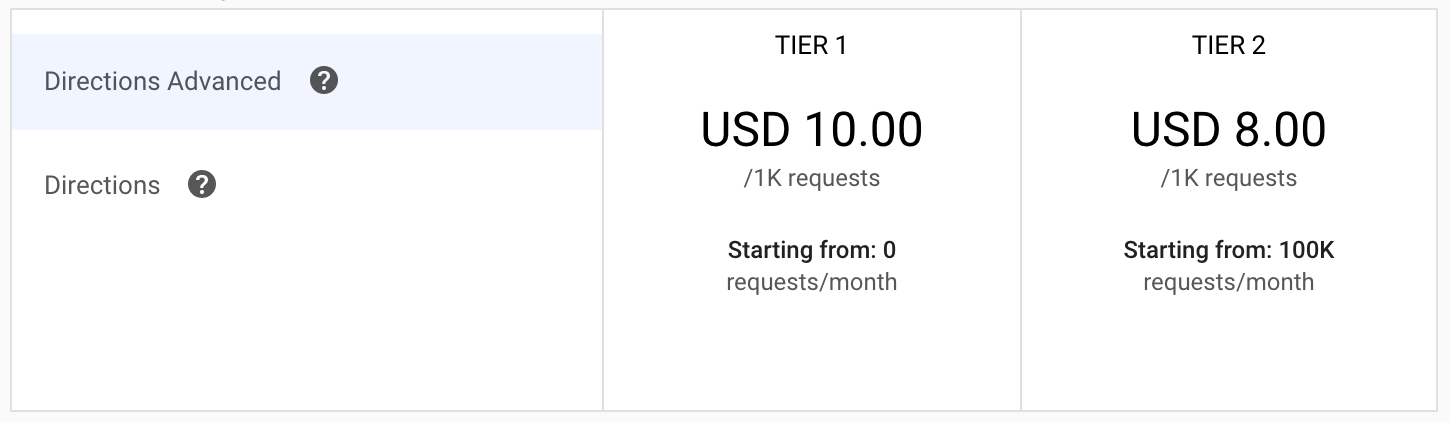
Find your Google Maps API Key
Next, while still logged into the GCP Console, go to the project you just created and enable the Directions API. Once that's done, go to the Google Maps Platform > Credentials page and generate a new API key (see this video for a step by step walkthrough). It should look something like this:
GOOGLE_API_KEY: AIzaSyBpGrzvyLhnNl_BJ8e7G6LLauQ_SFsHyTI
You can check that everything is set up correctly by going back to the project page and making sure that there's a green tick next to the Manage button on the Directions API page.
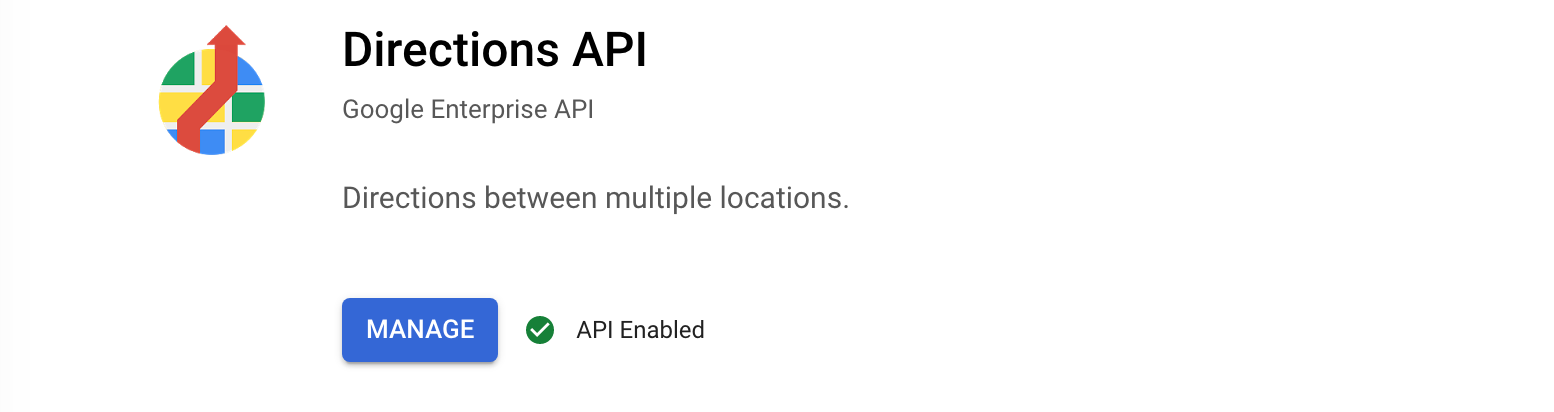
Make a Test Request
To make API requests, we are going to use an application called Postman. Postman gives us a user interface to authenticate with web services and save the results for future reference. Once you've downloaded and installed Postman, create a new GET
request and call this URL (remember to replace GOOGLE_API_KEY
with your own credentials).
For this test, we will retrieve the route going from (start) 1337 India St, San Diego, CA 92101, United States to (end) 1485 E St, San Diego, CA 92101, United States but ignore stops A, B, C and D. The first thing we need to do is encode the start and end addresses into ASCII so that it can be safely used in the API call.
Using a URL encoder, an address like "1337 India St, San Diego, CA 92101, United States" becomes "1485%20E%20St%2C%20San%20Diego%2C%20CA%2092101% 2C%20United%20States".
Now, let's see how a basic call to the Directions API is structured.
https://maps.googleapis.com/maps/api/directions/json?
origin={origin}
&destination={destination}
&key=GOOGLE_API_KEY
There are three parameters - {origin}, {destination} and GOOGLE_API_KEY. From the documentation, we are told that the API will return the most efficient route taken between origin and destination. Replace {origin} and {destination} with the encoded address strings for (start) and (end) and swap in your API key for GOOGLE_API_KEY. Here's what the final URL looks like:
https://maps.googleapis.com/maps/api/directions/json?origin=1337%20India%20St%2C%20San%20Diego%2C%20CA%2092101%2C%20United%20States&destination=1485%20E%20St%2C%20San%20Diego%2C%20CA%2092101%2C%20United%20States&key=GOOGLE_API_KEY
In Postman, click File > New > Http Request and choose GET
. Copy and paste the URL above into Postman and hit send.
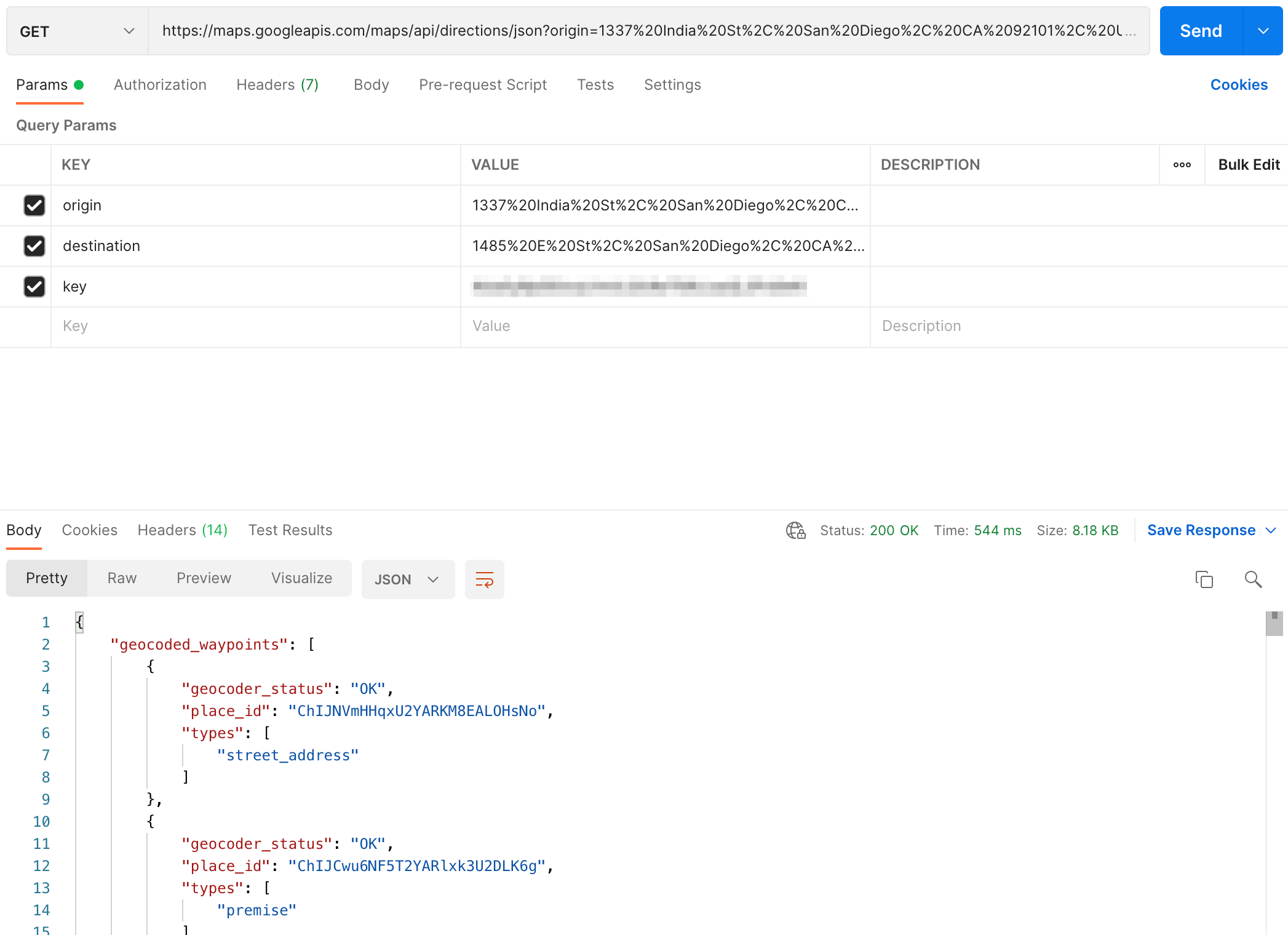
You'll get back a response that looks like this:
{
"geocoded_waypoints": [{
"geocoder_status": "OK",
"place_id": "ChIJNVmHHqxU2YARKM8EALOHsNo",
"types": [
"street_address"
]
},
{
"geocoder_status": "OK",
"place_id": "ChIJCwu6NF5T2YARlxk3U2DLK6g",
"types": [
"premise"
]
}
],
"routes": [{
"bounds": {
"northeast": {
"lat": 32.7194027,
"lng": -117.150393
},
"southwest": {
"lat": 32.7147013,
"lng": -117.167415
}
},
"copyrights": "Map data ©2021 Google",
"legs": [{
"distance": {
"text": "1.3 mi",
"value": 2146
},
"duration": {
"text": "7 mins",
"value": 438
},
"end_address": "1485 E St, San Diego, CA 92101, USA",
"end_location": {
"lat": 32.7147013,
"lng": -117.1507166
},
"start_address": "1337 India St, San Diego, CA 92101, USA",
"start_location": {
"lat": 32.7194027,
"lng": -117.1674044
},
"note": {
"comment": "...Additional results shortened in this example..."
},
"traffic_speed_entry": [],
"via_waypoint": []
}],
"overview_polyline": {
"points": "goufEfgcjUtBBCcJ@sDAmD@iDAiECcMCsG?gHAuFrECtCAn@?@U?k@AoA@kABqB?yAB_CAqD?mDA_C?Ib@?nCAhDAdA??`A"
},
"summary": "W A St",
"warnings": [],
"waypoint_order": []
}],
"status": "OK"
}
The most important parts of the response are the start_address
, start_location
, end_address
, end_location
and overview_polyline
. The start and end location fields give us the GPS coordinates that our driver starts from and ends at, while the polyline shows us the exact route taken. To decode this polyline, you need to use Google's polyline decoder library to convert the string "goufEfgcjUtBBCcJ@sDAmD@iDAiECcMCsG?gHAuFrECtCAn@?@U?k@AoA@kABqB?yAB_CAqD?mDA_C?Ib@?nCAhDAdA??`A" into an array of latitude and longitude pairs.
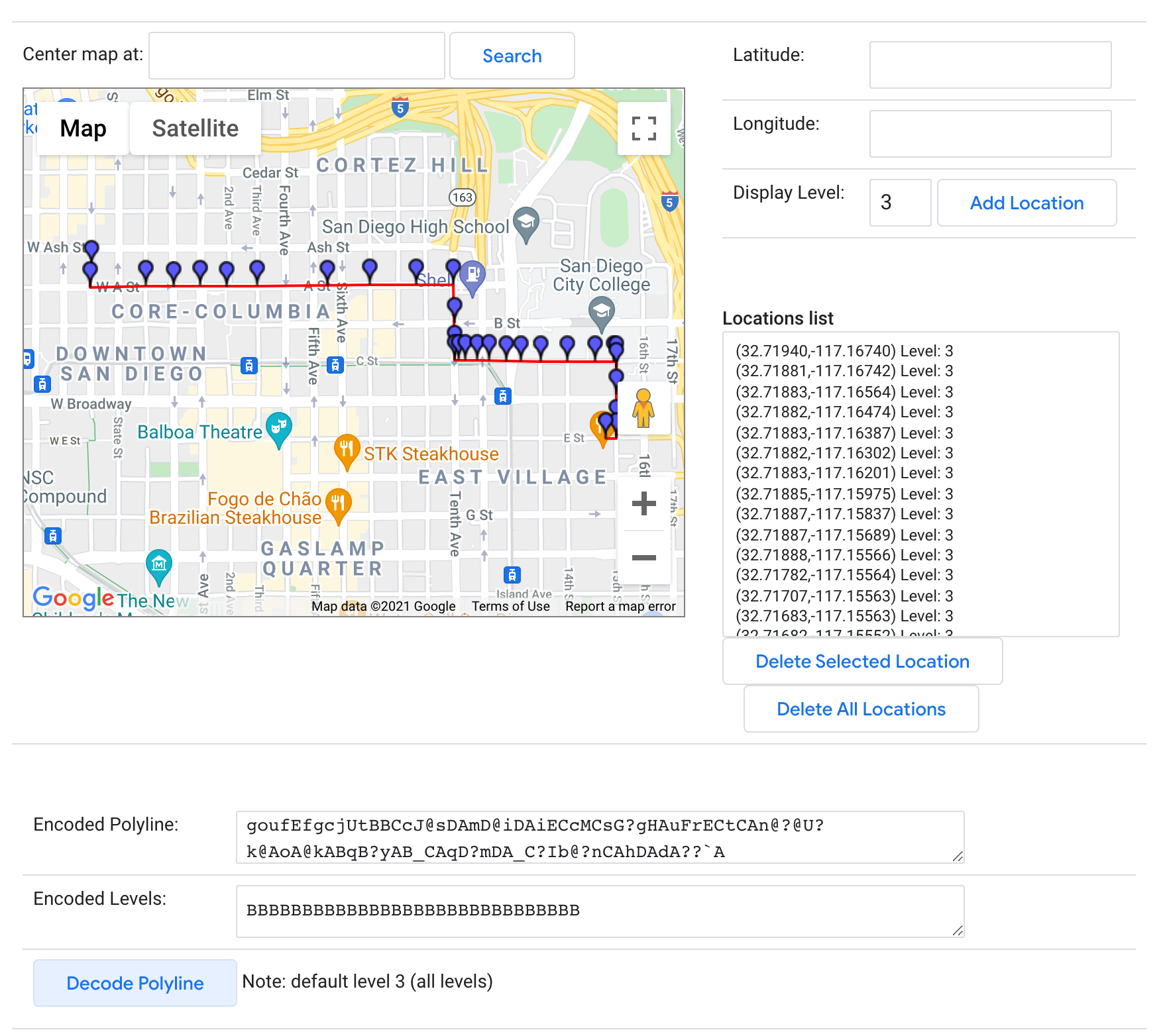
With this information, it's simple to join the start_location
and end_location
coordinates with the decoded overview_polyline
string to display your route on a map.
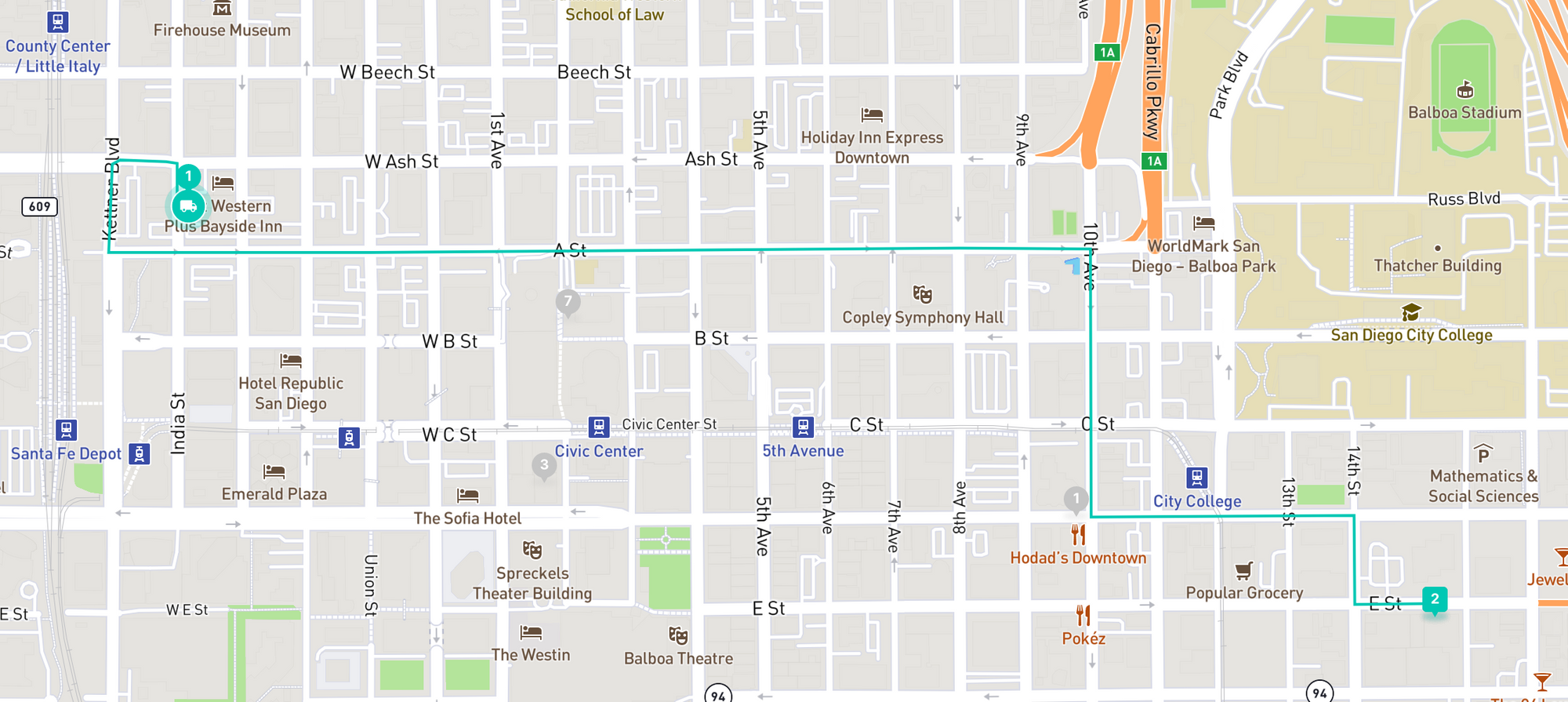
Retrieving the route between our start and end points is a great first step, but for this to be useful, we need to add delivery stops to the route and make sure these stops are ordered optimally to minimize drive time. My next post shows you how to modify your API call to do this.
Next: Part 3: Optimizing a Route with the Google Maps Directionxs API